Authentication
When a payment service provider registers with Peach Payments, they receive the following parameters for authentication:
- Client ID
- Client secret
Authentication consists of two categories:
- Authentication for inbound calls: API calls from the payment service provider to the Partner API.
- Authentication for outbound calls: API calls from the Partner API to the payment service provider.
Inbound call authentication
Before making calls to the Partner API, payment service providers must get an access token from the authentication service.
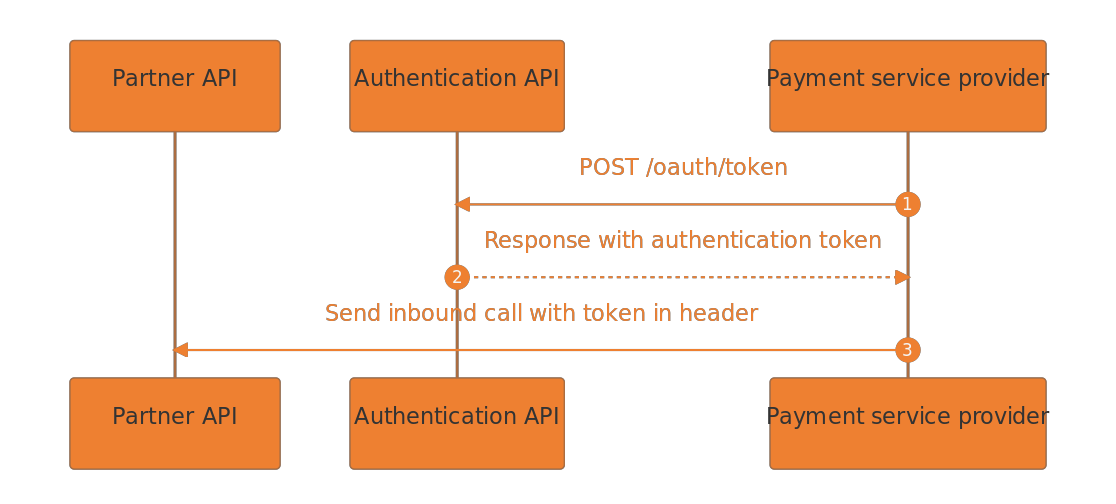
Inbound authentication flow.
- Make a POST
{peach-auth-service}/api/oauth/token
request to the Authentication API with your client ID and client secret to generate an access token.
curl --location --request POST '{peach-auth-service}/api/oauth/token' \
--header 'content-type: application/json' \
--data-raw
'{
"clientId": "{{app_client_id}}",
"clientSecret": "{{app_client_secret}}"
}'
- The Authentication API responds with an access token.
{
"access_token": "<access token>",
"expires_in": "<token expiry duration in seconds>",
"token_type": "Bearer"
}
- Make inbound API calls to the Partner API with the access token in the authorization header, for example,
Authorization: Bearer {access_token}
.
Notes
- The access token from step 2 can be reused for multiple API calls.
- When the token expires, you need to generate a new one.
Outbound call authentication
For calls that Peach Payments sends to the payment service provider, we offer optional token validation through the Python Partner Library or directly using the Authentication API.
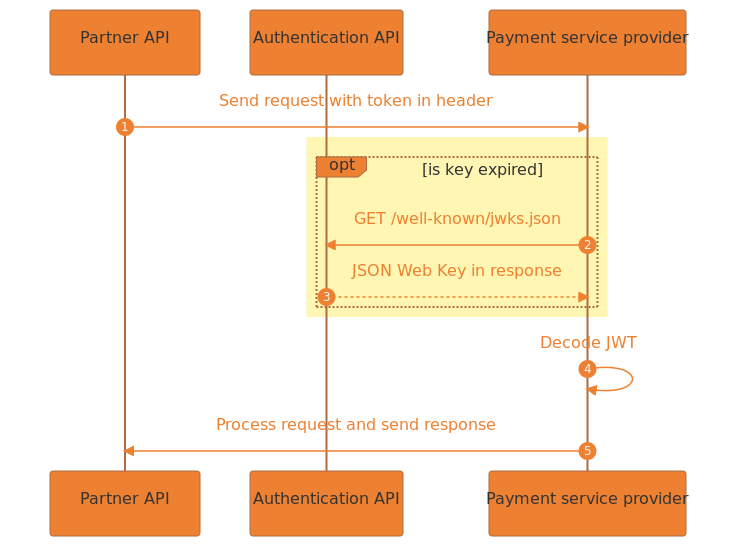
Outbound authentication flow.
- The Partner API sends an API request to the payment service provider.
If this is the first request or if the key has expired:
2. The payment service provider sends a GET{peach-auth-service}/.well-known/jwks.json
request to the Authentication API.
3. The Authentication API responds with the JSON Web Key.
{
"keys": [
{
"alg": "RS256",
"kty": "RSA",
"use": "sig",
"exp": "1652103166",
"n": "<public key modulus>",
"e": "<public key exponent>"
}
]
}
- The payment service provider decodes the JSON Web Key to authenticate the request. Ensure that you store the signing key in memory so that you only need to request a new one after it has expired.
- The payment service provider processes the request and sends a response back to the Partner API.
If you're using Python please use our peachpayments-partner library to reduce the code complexity.
🔑
Payment service provider - authenticate outbound requests
Open Recipe
Updated over 1 year ago